A General Note about Coding Interviews
These coding interview algorithm problems aren’t necessarily the best reflection of how you’ll perform at your job, but the vast majority of companies use them in their technical interviews. These challenges, at the very least, indicate your dedication to studying, your ability to comprehend complex abstractions, and your ability to think on your feet.
As long as companies still use these types of problems, you’ll need to prepare for them.
Problem Source / Inspiration
Glassdoor
Asked by: Facebook
Problem Description
Problem Solution
As always, let’s build our mental model. To do this, sometimes I like to create an illustration to visualize what is happening, especially for more complicated problems. So, let’s take a look.
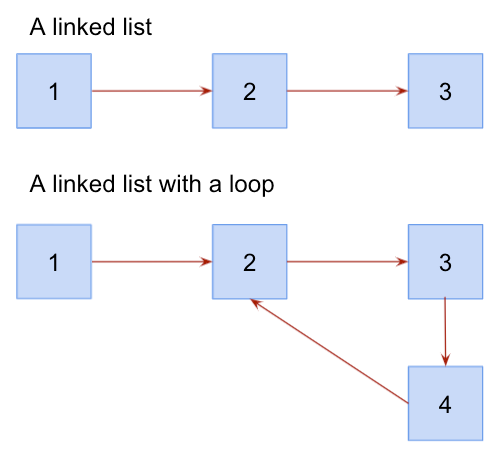
Typically when we are traversing through a linked list, we terminate our traversal when there are no more nodes to visit. In the case of the linked list with a loop, you will always have another node to visit because every node is pointing to some other node.
The key to solving this problem is to understand your termination conditions. Let’s dive deeper with some code.
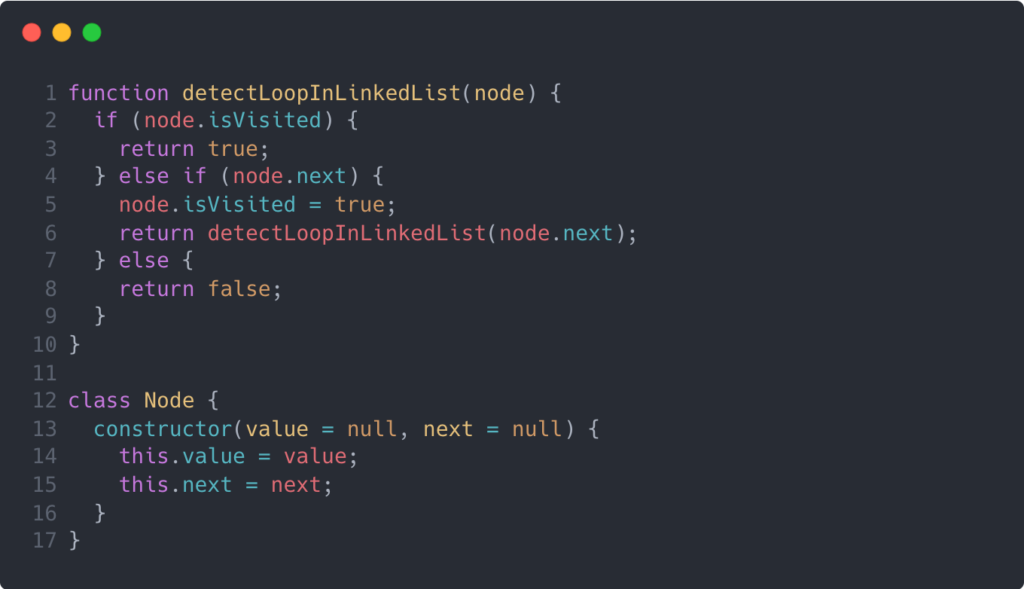
Notice that I’ve created a Node
class to help construct individual nodes. I’ve used it to create a linked list to test out my solution, and you should, too. If you’re new to linked lists, please check out this article I wrote that walks you through the process.
The solution ends up only being 10 lines of clear, legible code. I use a recursive solution, where I have two base cases or “terminating conditions.” The first base case terminates if I have encountered a node that I have previously visited. The other base case terminates if we have run out of nodes to traverse.
The recursive case in my solution is simple, but it’s the part that allows this solution to be O(1) space complexity. As seen on lines 5 and 6, I modify the current node to add a property isVisited
and assign it the value of true
. I then continue along with my traversal by invoking our function recursively and passing in the next node in the linked list. This way, if I do run into a node that I previously visited, we’ll be able to see that and terminate our recursive loop.
That’s it? Yup, that’s it. Sometimes these types of toy problems are just about being familiar with patterns. Interviewers are often trying to provide you with algorithm problems that you haven’t seen before. To do so, they combine problems, add slight twists to classic problems, or just simply reword them. It’s important to get a wide breadth of problems under your belt, so you can be familiar with several different patterns and their time and space complexities.
Conclusion
Once you grasp recursion and data structure traversal using recursion, this problem will appear fairly simple. And as with most problems, there are multiple ways to design this algorithm. It’s up to you to understand the benefits and drawbacks of each one.
Try solving this iteratively with a while
loop instead of using recursion. Or, what if your proctor informs you that you may not modify the nodes (i.e. do not add an isVisited
property to the nodes), but you can use more memory? Can you think of a more “brute force” solution using a hash map?
Michael has a diverse background in software engineering, mechanical engineering, economics, and management. After a successful career in mechanical engineering, Michael decided to become a software engineer and attended the Hack Reactor coding bootcamp. Michael enjoys writing about his experiences and helping others start their journies in software development.